Summary
Here is a quick little microservice I wrote as a KRL rule to ensure that the vehicle has all the subscriptions it needs and fix them if need be. The simplicity illstrates why a microservices approach holds such promise for more loosely coupled systems.
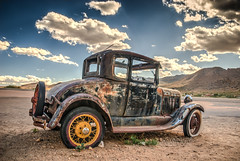
Last week I wrote about how Fuse uses a microservices architecture and the benefits that such an architecture provides. This morning I was faced with a problem that the microservices approach solved handily, so I thought I'd write it up as an example.
Fuse uses webhooks (we think of them as event channels) to receive notifications that a vehicle has started or stopped, has a low battery, etc. If these subscriptions aren't set up for a vehicle nothing works. Most noticably trips aren't recorded. Alex, who's working on the Fuse app, wasn't seeing any trips from his truck. Sure enough, when I checked, it had no Fuse event subscriptions.
I could have just reinitialized Alex's truck, but I figured if it happened to Alex then it's likely to happen to other people, so I created a simple microservice (i.e. KRL rule) that checks the number of subscriptions and if it's lower than the expected number, reinitializes them.
rule check_subscriptions { select when fuse subscription_check pre { vid = carvoyant:vehicle_id(); my_subs = carvoyant:getSubscription(vid); should_have = required_subscription_list.length(); } if(my_subs.length() < should_have) then { send_directive("not enough subscriptions") with my_subscriptions = my_subs and should_have = should_have } fired { log ">>>> vehicle #{vid} needs subscription check"; raise fuse event need_initial_carvoyant_subscriptions; } else { log ">>>> vehicle #{vid} has plenty of subscriptions"; } }
The rule uses a pre-existing function to get the current subscriptions and compares the length of that result to the number we should have. If there aren't enough the rule fires and raises the
fuse:need_initial_carvoyant_subscriptions
.
This is a really simple rule. One reason is because it makes use of other services that already exist. There's already a working function for getting the current subscriptions for a vehicle. There's already another service (or rule), called initialize subscriptions
, that sets up the subscriptions when they're needed.
Another reason the rule is simple is because it doesn't have to figure out which subscriptions are missing and limit itself to only initializing those. If any are missing, it asks for them all. That's because the initialize subscriptions
rule is idempotent. You can run it as many times as you like without messing anything up. Of course, I'd rather not put that load on the system if I don't have to, so the check_subscriptions
rule checks if something needs to be done before it raises the event.
The primary point is that the microservice architecture is loosely coupled and so setting up a service like this is easy. There's very little code, it makes use of other services, and it's unlikely to break anything. I wired it into the system by raising the event it looks for, fuse:subscription_check
when the vehicle profile is updated. That seems like a good compromise between over checking and user control.